iOSアプリにAdMobで広告を表示させる
自分で作成するiOSアプリに広告を仕組む方法です。
Googleの「AdMob」というものを使います。
作業フロー
下記の手順です。
- AdMobアカウント作成
- 広告ユニットを作成
- CocoaPodsをインストール
- Podfileを生成
- Podfileを編集
- AppDelegateにアプリIDを設定
- frameworkをプロジェクトに追加
- 広告を表示したいViewにコードを追加
AdMobアカウント作成
https://admob.google.com/intl/ja/home/
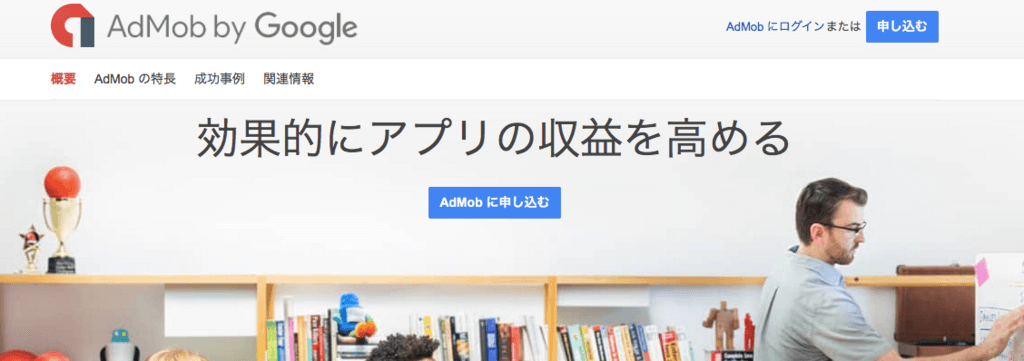
「AdMobに申し込む」をクリック。
Google AdSenseアカウントがあればそれを使えるので、簡単。なければここで作ることになると思う。
広告ユニットを作成
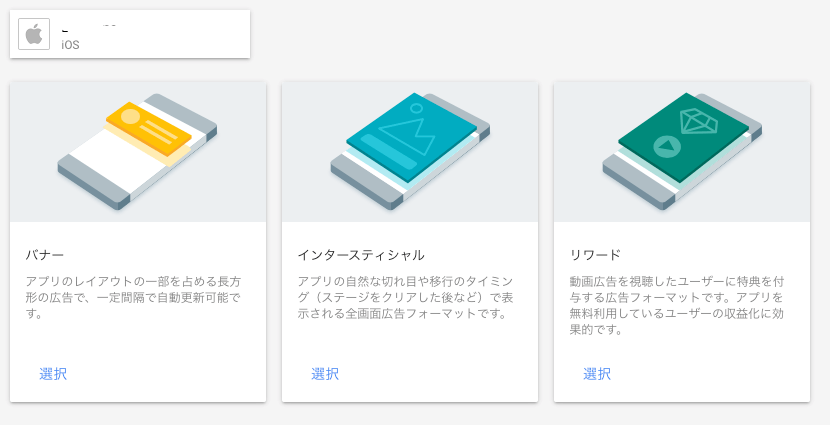
ぽちぽちと選ぶだけ。 ここではバナーを選んだ。
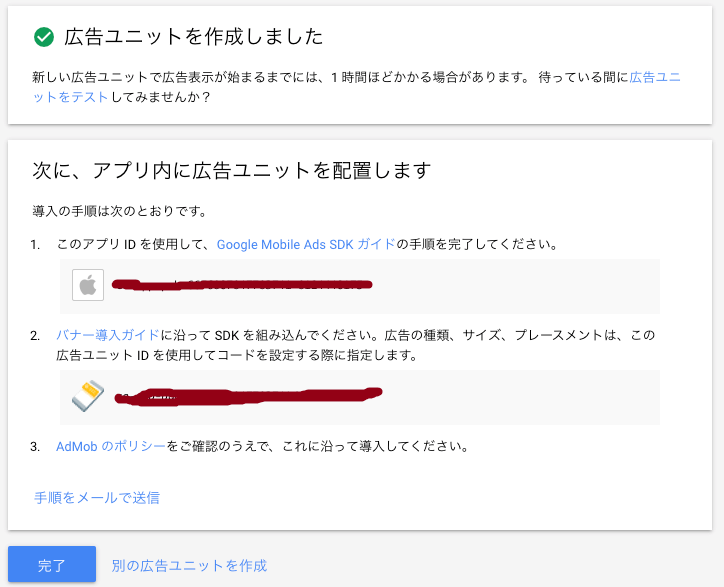
作成後の画面。
CocoaPodsをインストール
Google Mobile Ads SDKなるものを、自分のプロジェクトに組み込むための手順。
「CocoaPods」というライブラリ管理ツールを使う。
ターミナルを立ち上げ、コマンドを実行。
$ sudo gem install cocoapods
そのあと、下のコマンドを実行。
$ pod setup
しばらく待って終わり。
Podfileを生成
ターミナル上で、目的のプロジェクトまで移動。(プロジェクトファイル .xcodeprojがあるところまで移動)
その後、下記のコマンドを実行。
$ pod init
すると、「Podfile」というファイルが生成される。
Podfileを編集
下記の一行をPodfileに追加。
pod 'Google-Mobile-Ads-SDK'
その後、下記のコマンドを実行。実行前にXcodeは閉じること。
$ pod install --repo-update
AppDelegateにアプリIDを設定
ここからはXcode上の作業。
func application に下記の一行を追加。(GADMobileAds.configure ってやつ)
import GoogleMobileAds
…
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
func application(_ application: UIApplication,
didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool {
// Initialize the Google Mobile Ads SDK.
// Sample AdMob app ID: ca-app-pub-3940256099942544~1458002511
GADMobileAds.configure(withApplicationID: "YOUR_ADMOB_APP_ID")
return true
}
}
とりあえずテスト用のIDにしておく。
GADMobileAds.configure(withApplicationID: "ca-app-pub-3940256099942544~1458002511")
frameworkをプロジェクトに追加
このままだとリンカでたぶんエラーが出る。frameworkをプロジェクトに追加。
プロジェクトを選択し、「General」タブの下の方にある。「+」ボタンを押し、下記のようになるように一個一個追加していく。
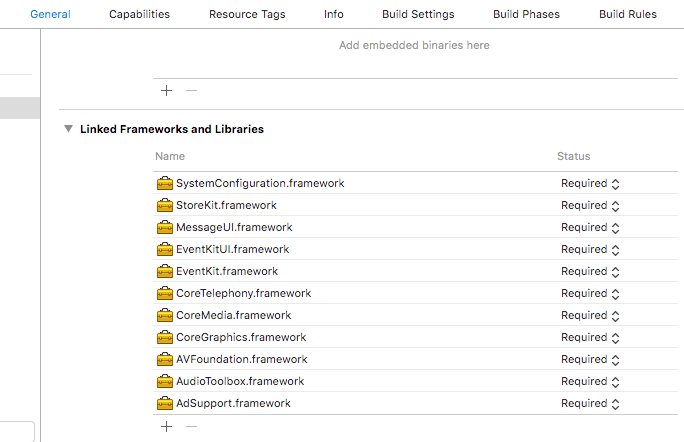
「podホニャララ」みたいなフォルダが勝手に追加されていたが、それがエラーの原因だったりしたので消したりした。
もしエラーが出てたら、この辺に不要なフォルダがあるかもしれないので疑う。
広告を表示したいViewにコードを追加
広告を表示したいViewのViewDidLoad()を、下記のように編集。
このIDはテスト用。
override func viewDidLoad() {
super.viewDidLoad()
// for AdMob
bannerView = GADBannerView(adSize: kGADAdSizeBanner)
bannerView.adUnitID = "ca-app-pub-3940256099942544/2934735716"
bannerView.rootViewController = self
bannerView.delegate = self
bannerView.load(GADRequest())
addBannerViewToView(bannerView)
}
addBannerViewToViewはこちら↓。
デバイスのサイズによって、勝手に広告サイズを調整してくれます。(Smart Bannerというのだそうな)
func addBannerViewToView(_ bannerView: GADBannerView) {
bannerView.translatesAutoresizingMaskIntoConstraints = false
view.addSubview(bannerView)
if #available(iOS 11.0, *) {
// In iOS 11, we need to constrain the view to the safe area.
positionBannerViewFullWidthAtBottomOfSafeArea(bannerView)
}
else {
// In lower iOS versions, safe area is not available so we use
// bottom layout guide and view edges.
positionBannerViewFullWidthAtBottomOfView(bannerView)
}
}
// MARK: - view positioning
@available (iOS 11, *)
func positionBannerViewFullWidthAtBottomOfSafeArea(_ bannerView: UIView) {
// Position the banner. Stick it to the bottom of the Safe Area.
// Make it constrained to the edges of the safe area.
let guide = view.safeAreaLayoutGuide
NSLayoutConstraint.activate([
guide.leftAnchor.constraint(equalTo: bannerView.leftAnchor),
guide.rightAnchor.constraint(equalTo: bannerView.rightAnchor),
guide.bottomAnchor.constraint(equalTo: bannerView.bottomAnchor)
])
}
func positionBannerViewFullWidthAtBottomOfView(_ bannerView: UIView) {
view.addConstraint(NSLayoutConstraint(item: bannerView,
attribute: .leading,
relatedBy: .equal,
toItem: view,
attribute: .leading,
multiplier: 1,
constant: 0))
view.addConstraint(NSLayoutConstraint(item: bannerView,
attribute: .trailing,
relatedBy: .equal,
toItem: view,
attribute: .trailing,
multiplier: 1,
constant: 0))
view.addConstraint(NSLayoutConstraint(item: bannerView,
attribute: .bottom,
relatedBy: .equal,
toItem: bottomLayoutGuide,
attribute: .top,
multiplier: 1,
constant: 0))
}
あと、下記の関数は、広告に対して何かイベントが起きた時に呼ばれるやつ。
いつか使うかも?使わないかも?
// Called when an ad request loaded an ad.
func adViewDidReceiveAd(_ bannerView: GADBannerView) {
print(#function)
}
// Called when an ad request failed.
func adView(_ bannerView: GADBannerView, didFailToReceiveAdWithError error: GADRequestError) {
print("\(#function): \(error.localizedDescription)")
}
// Called just before presenting the user a full screen view, such as a browser, in response to
// clicking on an ad.
func adViewWillPresentScreen(_ bannerView: GADBannerView) {
print(#function)
}
// Called just before dismissing a full screen view.
func adViewWillDismissScreen(_ bannerView: GADBannerView) {
print(#function)
}
// Called just after dismissing a full screen view.
func adViewDidDismissScreen(_ bannerView: GADBannerView) {
print(#function)
}
// Called just before the application will background or terminate because the user clicked on an
// ad that will launch another application (such as the App Store).
func adViewWillLeaveApplication(_ bannerView: GADBannerView) {
print(#function)
}
実行例 (テスト用ID)
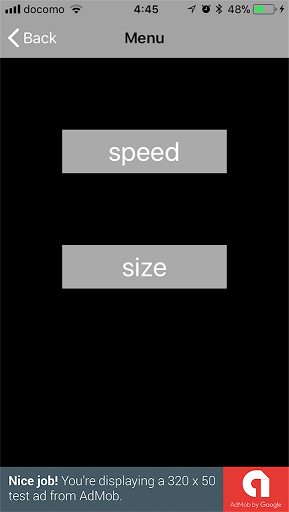
こんな感じになりました。Nice job!
あとは、自分のIDに置き換えてテストすれば完成。